Greedy Algorithms Interview Questions
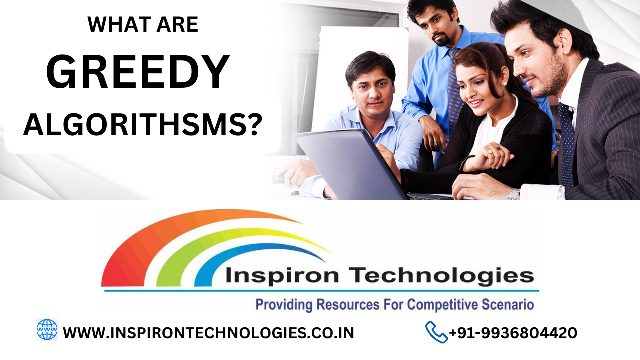
What are Greedy Algorithms?
Greedy algorithms are a class of algorithms that make a series of choices by selecting the best immediate option at each step in the hope of finding a global optimum. They are used in various optimization problems where the goal is to find the best solution by making a sequence of choices. Greedy algorithms work well for problems where choosing the locally optimal solution at each step leads to the globally optimal solution.
Here's a detailed explanation of how greedy algorithms work:
Problem Definition: To apply a greedy algorithm, you first need to define the problem you're trying to solve as an optimization problem, where you aim to either maximize or minimize some objective function or criteria. The problem should have the property that it can be broken down into a sequence of choices.
Greedy Choice Property: The key characteristic of a greedy algorithm is the greedy choice property, which means that at each step, the algorithm makes the best choice available at that moment without considering the consequences of that choice on future steps. In other words, it selects the locally optimal solution.
Optimal Substructure: Another important property required for a problem to be solved using a greedy approach is optimal substructure. This means that the solution to the problem can be constructed from solutions to subproblems. In other words, solving part of the problem independently will contribute to solving the entire problem optimally.
Algorithm Execution: Greedy algorithms typically follow a straightforward pattern:
Initialize the solution to an empty or trivial solution.
Iterate through the available choices, and at each step, choose the best option according to a specific criterion.
Update the solution with the chosen option.
Repeat this process until the problem is solved.
Termination: Greedy algorithms terminate when they have made all necessary choices and constructed a complete solution.
Optimality Analysis: Once the greedy algorithm terminates, it is essential to prove that the solution it found is indeed globally optimal. This typically involves showing that the locally optimal choices made at each step do not lead to a suboptimal result for the overall problem.
It's important to note that not all optimization problems can be solved using a greedy algorithm. Greedy algorithms are most effective when the problem exhibits the greedy choice property and optimal substructure. In some cases, making locally optimal choices at each step does not guarantee a globally optimal solution.
Common examples of problems that can be solved with greedy algorithms include:
Minimum Spanning Tree: Finding the minimum spanning tree of a graph.
Shortest Path: Finding the shortest path in a graph (e.g., Dijkstra's algorithm).
Fractional Knapsack Problem: Maximizing the value of items placed in a knapsack with a weight constraint.
Huffman Coding: Constructing a variable-length prefix coding for data compression.
In these examples, making the best immediate choice at each step leads to an optimal solution. However, in more complex problems, dynamic programming or other techniques may be required to find the global optimum.
Explain what a greedy algorithm is. Provide an example.
Explanation: In this question, the interviewer is testing your fundamental understanding of greedy algorithms. Begin by defining a greedy algorithm as one that makes locally optimal choices at each step in the hope of finding a global optimum. Provide a simple example, such as the coin change problem, where you aim to make change with the fewest coins.
What is the greedy choice property? How does it affect the decision-making process in a greedy algorithm?
Explanation: The greedy choice property is a key characteristic of greedy algorithms. It states that at each step, a greedy algorithm makes the best choice available at that moment without considering the consequences of that choice on future steps. Explain that this property ensures that the algorithm selects the locally optimal solution at each step.
Can you give an example of a problem where a greedy algorithm will not produce an optimal solution?
Explanation: Discuss a classic example like the "Traveling Salesman Problem" where a greedy approach may not guarantee the globally optimal solution. Explain that in such cases, the locally optimal choices do not necessarily lead to the best overall solution, and additional techniques like dynamic programming might be needed.
What are the key components that a greedy algorithm typically consists of?
Explanation: Explain that a greedy algorithm generally consists of the following components:
A set of choices at each step.
A selection criterion to determine the best choice.
An objective function that defines the optimization goal.
A solution construction mechanism.
Describe the algorithm for solving the Fractional Knapsack Problem using a greedy approach.
Explanation: Walk through the steps of the Fractional Knapsack Problem, where you have a set of items with weights and values, and you want to maximize the total value in a knapsack with a weight constraint. Explain that the greedy choice is to take the item with the highest value-to-weight ratio first, and repeat this process until the knapsack is full.
What is the Huffman coding algorithm, and how does it work?
Explanation: Explain that Huffman coding is a variable-length prefix coding used for data compression. Describe how it constructs a binary tree where characters with higher frequencies are closer to the root, resulting in shorter codes for frequently occurring characters and longer codes for less frequent characters. Emphasize the greedy choice of merging the two lowest frequency characters at each step.
Compare Dijkstra's algorithm with Bellman-Ford algorithm. When would you prefer one over the other?
Explanation: Dijkstra's algorithm and Bellman-Ford algorithm are used for finding the shortest path in a graph. Discuss their differences and explain that Dijkstra's algorithm is preferred when all edge weights are non-negative, while Bellman-Ford is more versatile and can handle graphs with negative weights but detects negative weight cycles.
Can you describe an application of a greedy algorithm in real-life situations, such as scheduling or optimization problems?
Explanation: Provide an example of a real-world problem that can be solved using a greedy algorithm. For instance, you can mention task scheduling, where you aim to minimize the total completion time, and show how a greedy algorithm can efficiently solve it.
These questions test your understanding of the principles behind greedy algorithms, your ability to apply them to real problems, and your knowledge of situations where a greedy approach might not be suitable. It's essential to provide clear explanations and, when applicable, discuss the limitations of greedy algorithms.
Explain the concept of the "Interval Scheduling Problem" and how a greedy algorithm can be used to solve it.
Explanation: The Interval Scheduling Problem involves scheduling a set of tasks with start and end times to maximize the number of non-overlapping intervals. Describe how a greedy algorithm selects the task that ends earliest and is compatible with the current schedule, iterating until no more compatible tasks can be added. This approach ensures maximum task scheduling.
What is the "Activity Selection Problem"? Can you describe a greedy algorithm to solve it?
Explanation: The Activity Selection Problem involves selecting the maximum number of non-overlapping activities from a set of activities with start and finish times. Explain the greedy approach of selecting the activity with the earliest finish time, as long as it doesn't overlap with the previously selected activity. This ensures a maximum set of non-overlapping activities.
Discuss the concept of the "Coin Change Problem" and explain how a greedy algorithm can be used to make change with the fewest coins.
Explanation: The Coin Change Problem involves making change for a given amount using the fewest coins. Explain the greedy choice of selecting the largest denomination coin that is less than or equal to the remaining amount. Continue this process until the remaining amount becomes zero. Mention that this approach works when coin denominations are in a specific order (e.g., sorted in descending order).
When using a greedy algorithm, what precautions should be taken to ensure that it indeed produces the optimal solution?
Explanation: Discuss the precautions to be taken with greedy algorithms. Explain the need for a clear greedy choice property and optimal substructure in the problem, as well as the importance of proving the algorithm's optimality. Emphasize the need to verify that locally optimal choices at each step lead to a globally optimal solution.
Can you provide an example where a greedy algorithm might be applied to solve a problem in a business or industry context?
Explanation: Share a practical business or industry scenario, such as optimizing delivery routes for a delivery service. Explain how a greedy algorithm can be employed to efficiently schedule deliveries in a way that minimizes travel time and costs.
Explain how a greedy algorithm can be used to find the minimum spanning tree in a graph.
Explanation: Describe how Kruskal's or Prim's algorithm works to find the minimum spanning tree. Mention that the greedy choice is to add edges with the smallest weights while ensuring that no cycles are formed. This eventually results in a tree that spans all the vertices with minimal total weight.
What is the "Knapsack Problem," and how does it differ from the "Fractional Knapsack Problem" regarding the use of greedy algorithms?
Explanation: Differentiate between the Knapsack Problem, where items must be either taken or left, and the Fractional Knapsack Problem, where fractions of items can be selected. Explain that a greedy algorithm can be used to solve the Fractional Knapsack Problem, as it chooses fractions of items based on their value-to-weight ratios.
These questions cover various aspects of greedy algorithms, including their applications, use cases, and precautions to ensure optimality. Preparing for these questions will help you demonstrate your understanding of greedy algorithms in an interview setting.
What is the "Greedy Algorithm for Egyptian Fractions," and how does it work?
Explanation: Explain the Egyptian fraction representation problem, where the goal is to represent a given fraction as the sum of unit fractions (fractions with a numerator of 1). Describe the greedy algorithm, which continually finds the largest unit fraction that is less than or equal to the remaining fraction until the fraction becomes 0. This approach always results in a finite representation using unit fractions.
Discuss the "Job Scheduling Problem" and explain how a greedy algorithm can be applied to optimize job scheduling.
Explanation: The Job Scheduling Problem involves scheduling a set of jobs with given processing times and deadlines to minimize the total penalty for missed deadlines. Explain how a greedy algorithm can work by sorting the jobs by deadlines and scheduling them in a way that minimizes penalty, usually by considering earliest deadlines first.
Can you provide an example of a problem where a greedy algorithm is more efficient than a dynamic programming approach?
Explanation: Compare the characteristics of greedy algorithms and dynamic programming. Give an example like the "Change-making problem" or "Activity Selection Problem" where a greedy approach is more efficient and simpler because it doesn't require solving subproblems or storing additional information.
Explain the "Candy Distribution Problem" and how a greedy algorithm can be used to distribute candies fairly among children.
Explanation: The Candy Distribution Problem involves distributing candies to children with different ratings while ensuring that children with higher ratings get more candies. Describe the greedy approach, which starts with equal candies for all children and iteratively adjusts the distribution based on children's ratings to achieve fairness.
What are the advantages and disadvantages of using greedy algorithms in problem-solving?
Explanation: Discuss the pros and cons of using greedy algorithms. Mention the advantages, such as simplicity and efficiency, as they often provide fast solutions. On the downside, mention the need for problem-specific proofs of optimality and the potential for making incorrect choices in some cases.
Explain the "Buy and Sell Stock" problem and how a greedy algorithm can be used to find the maximum profit.
Explanation: The Buy and Sell Stock problem involves finding the best times to buy and sell a stock to maximize profit. Describe the greedy approach of iterating through prices and tracking the minimum buying price and the maximum profit that can be obtained if selling at the current price. Explain that the algorithm captures the locally optimal choices to determine the global maximum profit.
Discuss a situation where a greedy algorithm may lead to suboptimal results due to a particular problem's characteristics.
Explanation: Provide an example, such as a case where greedy algorithms don't work, like the "Travelling Salesman Problem" or problems with non-greedy solutions. Emphasize that the key is recognizing when the greedy approach might not apply due to the problem's unique constraints.
These additional questions delve deeper into various aspects of greedy algorithms, including specific problem scenarios and their pros and cons. Preparing for these questions will help you demonstrate a more comprehensive understanding of greedy algorithms in an interview context.
Explain the "Minimum Platforms Required" problem in the context of train scheduling and describe how a greedy algorithm can be used to solve it.
Explanation: The Minimum Platforms Required problem involves scheduling trains' arrival and departure times to minimize the number of platforms needed. Discuss the greedy algorithm approach, which sorts the events by time, keeps track of trains on platforms, and determines the minimum platforms required.
What is Huffman coding, and how does it achieve data compression through a greedy algorithm approach?
Explanation: Describe Huffman coding as a variable-length prefix coding used for data compression. Explain that it constructs a binary tree where frequently occurring characters have shorter codes. The greedy choice is to merge the two lowest frequency characters at each step, creating an optimal encoding.
Can you provide an example of a problem where a greedy algorithm might have multiple solutions, and explain how to select the best one?
Explanation: Offer an example like the "Job Scheduling Problem" with varying penalties for missed deadlines. Explain that when multiple solutions are possible, you might need additional criteria or heuristics to select the best one, such as minimizing penalties or maximizing profits.
Describe the "Prim's Algorithm" for finding the minimum spanning tree in a graph. How does it make greedy choices in building the tree?
Explanation: Explain that Prim's Algorithm starts with an arbitrary vertex and repeatedly adds the edge with the minimum weight that connects a vertex in the tree to one outside it. The greedy choice is to select the minimum-weight edge at each step, which ensures that the tree spans all vertices with the least total weight.
What are some practical scenarios where a greedy algorithm is particularly well-suited for solving real-world problems?
Explanation: Discuss practical applications where greedy algorithms are useful, such as task scheduling, job sequencing, and data compression. Explain that in these scenarios, making locally optimal choices often leads to globally optimal or near-optimal solutions.
Explain the "DAG Shortest Path" problem and how a greedy algorithm can be applied to find the shortest paths in a Directed Acyclic Graph (DAG).
Explanation: Describe the problem of finding the shortest paths in a DAG, where edge weights are non-negative. Explain the greedy approach of topological sorting to order the vertices and then relaxing edges in topological order, ensuring that you always consider the shortest path to each vertex.
Can you discuss a case where a greedy algorithm might be used to address a problem involving resource allocation in a constrained environment?
Explanation: Offer a real-world scenario like network bandwidth allocation or resource scheduling in cloud computing. Explain how a greedy algorithm can efficiently allocate resources, making locally optimal choices while adhering to constraints.
What are some common pitfalls when designing a greedy algorithm, and how can you mitigate them?
Explanation: Discuss potential issues like failing to prove optimality, choosing incorrect greedy criteria, or overlooking problem constraints. Explain that these pitfalls can be mitigated by carefully analyzing the problem, conducting proof by contradiction, and considering alternative greedy strategies.
These questions explore various aspects of greedy algorithms, including problem-solving applications, practical scenarios, and potential challenges. Preparing for these questions will help you demonstrate a deeper understanding of how to apply and analyze greedy algorithms in different contexts.
We hope that you must have found this exercise quite useful. If you wish to join online courses on Power BI, Tableau, AI, IOT, DevOps, Android, Core PHP, Laravel Framework, Core Java, Advance Java, Spring Boot Framework, Struts Framework training, feel free to contact us at +91-9936804420 or email us at aditya.inspiron@gmail.com.
Happy Learning
Team Inspiron Technologies
Leave a comment